Introduction
The Leap Motion Controller is a piece of hardware that captures the movement of human hands and fingers with unparalleled accuracy and low latency. With the traditional mesh viewer, users rotate, translate, and zoom the model using a 3-button mouse. In this tutorial, I utilize Leap Motion Controller instead of a typical mouse controller to control a 3D Mesh Viewer’s translation, scaling, rotation, stop, and reset features. I also wrote the 3D Mesh Viewer, which can open a 3D model file (the 3D mesh file will be parsed into a half-edge data structure in the software) and render 3D Mesh using the OpenGL module. Therefore, the program is a combination of 3D Mesh Viewer with Leap Motion control.
Demo video:
Detail on Interaction Style
In this part, I will introduce the detail of interaction style for my program. And, we need to know that both Leap Motion and OpenGL rendering window use right-handed coordinate system. The origin of Leap Motion is $(0, 0, 0)$.
Translation
- Only detection of left hand indicates the translation motion.
- Default left-hand palm position $(x, y, z) = (0, 200mm, 0)$ is predefined by code, if palm position is $(0, 200, 0)$, the mesh object won’t have any translation motion, all palm position will be compared with $(0, 200 ,0)$.
- If left hand is moving towards $+X/+Y/+Z$ direction, the mesh object will translate towards $+X/+Y/+Z$ direction, $-X/-Y/-Z$ direction also apply. It means that the mesh object will follow the left-hand motion to translate with same direction.
Scaling
- Detection of both hands indicates the scaling/zoom motion.
- Left-hand position is not considered in scaling condition; default height ($Y$) difference ($200mm$) between right-hand palm and leap motion is predefined by code, scale factor will be 1.0 if the height difference is 200; scale factor will be greater than 1.0 if the height difference is greater than 200; scale factor will be less than 1.0 if the height difference is less than 200.
Rotation
- Only detection of right hand indicates the rotation motion.
- Default right hand palm position $(x, y, z) = (0, 200mm, 0)$ is predefined by code, if palm position is $(0, 200, 0)$, the mesh object won’t have any rotation motion, all palm position will be compared with $(0, 200, 0)$.
- If right hand is moving towards $+X$ direction, the mesh object will rotate about $Z$ axis in counter-clockwise direction (view from $+Z$ region); If right hand is moving towards $-X$ direction, the mesh object will rotate about $Z$ axis in clockwise direction (view from $+Z$ region).
- If right hand is moving towards $+Z$ direction, the mesh object will rotate about $X$ axis in clockwise direction (view from $+X$ region); If right hand is moving towards $-Z$ direction, the mesh object will rotate about $X$ axis in counter-clockwise direction (view from $+X$ region).
- If right hand is moving towards $+Y$ direction, the mesh object will rotate about $Y$ axis in counter clockwise direction (view from $+Y$ region); If right hand is moving towards $-Y$ direction, the mesh object will rotate about $Y$ axis in clockwise direction (view from $+Y$ region).
Stop Motion
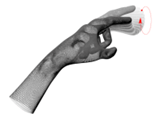
- If circle gesture is detected, program will stop the mesh motion, keep the current status and quit the current mode (translation/scale/rotation).
- For example, when you are using left hand to translate object, you can use one of left hand fingers to tracing a circle, then motion will stop; then you can use right-hand to scale object or use both hands to rotate object.
- If you want to change mode (translation/scale/rotation), it’s recommended to use circle gesture to keep current object status.
Reset Mesh Object
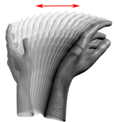
- If swipe gesture is detected anytime, program will stop the mesh motion, quit the current mode and reset mesh object status (back to original position, scale size and rotation).
Project Code
Requirement
- LeapSDK 2.3.1
- Qt 5.12, OpenGL function is provided by QtOpenGL Module, QGLWidget class in QtOpenGL is used to render OpenGL graphics.
import LeapSKD in Qt
Open the .pro file in Qt project directory, add the following code:
win32: {
message("Windows OS build")
LIBS += opengl32.lib
INCLUDEPATH += C:/LeapSDK/include
!contains(QMAKE_HOST.arch, x86_64) {
message("win x86 build")
LIBS += C:/LeapSDK/lib/x86/Leap.lib
} else {
message("win x86_64 build")
LIBS += C:/LeapSDK/lib/x64/Leap.lib
}
}
macx: {
message("macOS build")
INCLUDEPATH += /Users/zclin/Documents/LeapSDK/include
LIBS += -L/Users/zclin/Documents/LeapSDK/lib/ -lLeap
}
Include the following header in the corresponding header file:
#include "Leap.h"
using namespace Leap;
Code
You can get the full code here.