Update 2020: You can now directly call OpenCV C/C++ or Python API to use Pi Camera without any configuration now, just like use a normal USB webcam. Here is the sample code:
// Opencv needs to be installed on your Raspberry Pi
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
int main() {
VideoCapture cap(0); // if you only have 1 camera connected.
if (!cap.isOpened()) {
cout << "Cannot open camera\n";
return -1;
}
Mat frame;
while (true) {
bool ret = cap.read(frame); // or cap >> frame;
if (!ret) {
cout << "Error. Fail to receive frame.\n";
break;
}
......
// process frame
......
if (waitKey(1) == 'q') {
break;
}
}
return 0;
}
Now, using the Pi Camera in the method mentioned above is advised. But the below old way still works.
Normally OpenCV C/C++ only supports USB camera for Raspberry Pi. If you want to use OpenCV C/C++ with Pi Camera module, I have a nice guide for you.
Before we set the Pi Camera, you have to install OpenCV native library firstly, you can follow my previous guide.
I use the RaspiCam library from Rafael Muñoz Salinas (very good job, easy installation and fast speed), it provides C++ API for us, frame per second is almost 30.
Here are steps:
-
Plug the Pi camera into Raspberry Pi (I am using Raspberry Pi 2).
-
Download RaspiCam library into your Pi.
-
Install the library.
// uncompress the file
tar xvzf raspicam-0.1.3.tgz
// go to the library folder
cd raspicam-0.1.3
mkdir build
cd build
cmake ..
make
sudo make install
- Testing.
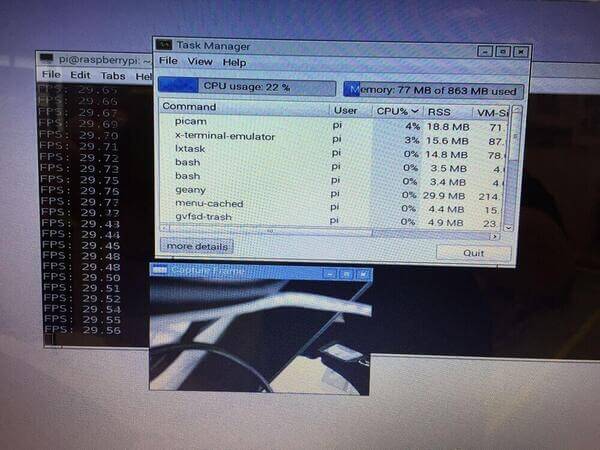
The library provides cmake to compile the program, but it’s not convenient to use for me, so I wrote makefile to compile the program. Here is the makefile:
LIBS = -I/usr/local/include/
CFLAGS = -lraspicam -lraspicam_cv -lopencv_core -lopencv_highgui
objects= main.o camera.o
picam: $(objects)
g++ $(objects) -o picam $(LIBS)$(CFLAGS)
main.o: main.cpp camera.h
g++ main.cpp -c
camera.o: camera.cpp camera.h
g++ camera.cpp -c
.PHONY: clean
clean:
rm picam $(objects)
The library provides some examples to us, I rewrote the sample code, actually we also can use OpenCV C API (I transfer cv::Mat to IplImage in sample code).
Here is sample code, download to your Raspberry Pi, make and run the program, FPS is ~29.7(CPU usage is 22%), amazing!
–END–